Why Handling Numbers in React is Hard: A Multi-Currency Challenge for E-Commerce
When building e-commerce applications in React, one of the most deceptively complex challenges is properly handling number inputs, especially when dealing with multiple currencies. What seems like a straightforward task—displaying and manipulating numbers—becomes surprisingly intricate when you consider formatting, validation, localization, and precision requirements.
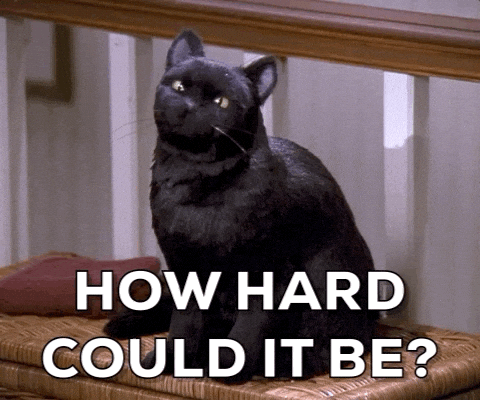
Let me walk you through the challenges and how specialized libraries can help solve them.
The Challenges of Number Input in JavaScript and React
1. JavaScript's Floating-Point Precision Issues
JavaScript stores numbers as 64-bit floating point values, which leads to infamous precision errors:
In an e-commerce context, these small errors can compound into significant accounting problems.
2. Formatting Differences Across Currencies
Different currencies have different formatting conventions:
- USD: $1,234.56
- EUR: €1.234,56
- JPY: ¥1,235 (no decimal places)
3. Input vs. Display Format
Users need to input numbers in a natural way, but the application needs to store and calculate with the raw numeric values.
4. Validation Requirements
Numbers need constraints like:
- Minimum/maximum values
- Decimal place limitations
- Preventing non-numeric characters
Solutions with Specialized Libraries
Using react-number-format
react-number-format provides a comprehensive solution for formatting and manipulating number inputs.
Here's a basic example for a price input:
This component handles:
- Currency-specific prefixes/suffixes
- Appropriate thousand and decimal separators
- Different decimal precision based on currency
Using @number-flow/react
@number-flow/react offers a more modern approach with features specifically designed for complex number handling scenarios.
Here's an example of implementing a multi-currency price input:
The @number-flow/react
library excels at:
- Consistent behavior across browsers and devices
- Handling cursor position intelligently when formatting
- Built-in accessibility features
- Efficient rendering optimizations
Advanced Usage: Currency Conversion Widget
Here's a more complex example showing a currency conversion widget:
Conclusion
Properly handling numeric inputs in React applications—especially in multi-currency e-commerce scenarios—is much more challenging than it initially appears. The complexities of number formatting, localization, validation, and JavaScript's inherent floating-point limitations make this a genuine problem space.
Libraries like react-number-format and @number-flow/react abstract away these complexities, providing robust, user-friendly solutions that handle:
- Currency-specific formatting
- Locale-aware separators
- Proper decimal precision
- Clean user input experience
- Underlying numeric value management
By leveraging these specialized tools, you can focus on building your e-commerce features rather than fighting with the intricacies of number representation and manipulation across different currencies.
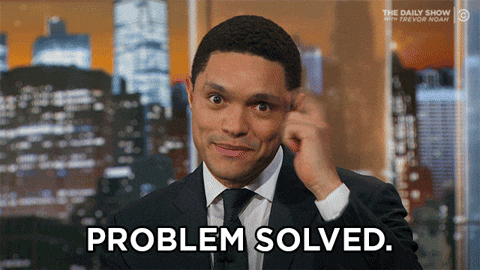